Here is the Python script to pass data to Appian Web API using username and password.
import requests
import json
# Set your Appian environment URL
APPIAN_ENV_URL = "https://my-appian.com"
# Set your Appian login credentials
APPIAN_USERNAME = "my-username"
APPIAN_PASSWORD = "my-password"
# Create a requests session
session = requests.Session()
# Authenticate to Appian
auth_url = APPIAN_ENV_URL + "/suite/api/authentication/login"
auth_headers = {
"Content-Type": "application/json"
}
auth_payload = {
"username": APPIAN_USERNAME,
"password": APPIAN_PASSWORD
}
auth_response = session.post(auth_url, headers=auth_headers, data=json.dumps(auth_payload))
# Get the authentication token
auth_token = auth_response.json()["token"]
# Set the authentication token in the session headers
session.headers.update({"Authorization": "Bearer " + auth_token})
# Set the URL of the Appian web API you want to call
webapi_url = APPIAN_ENV_URL + "/suite/api/webapi/my-webapi"
# Set the data you want to pass to the Appian web API
webapi_payload = {
"data": "my-data"
}
# Call the Appian web API
webapi_response = session.post(webapi_url, data=json.dumps(webapi_payload))
# Check the response status code
if webapi_response.status_code == 200:
print("Web API call successful!")
else:
print("Web API call failed: {}".format(webapi_response.status_code))
# Close the requests session
session.close()
Don’t forget to close the session.
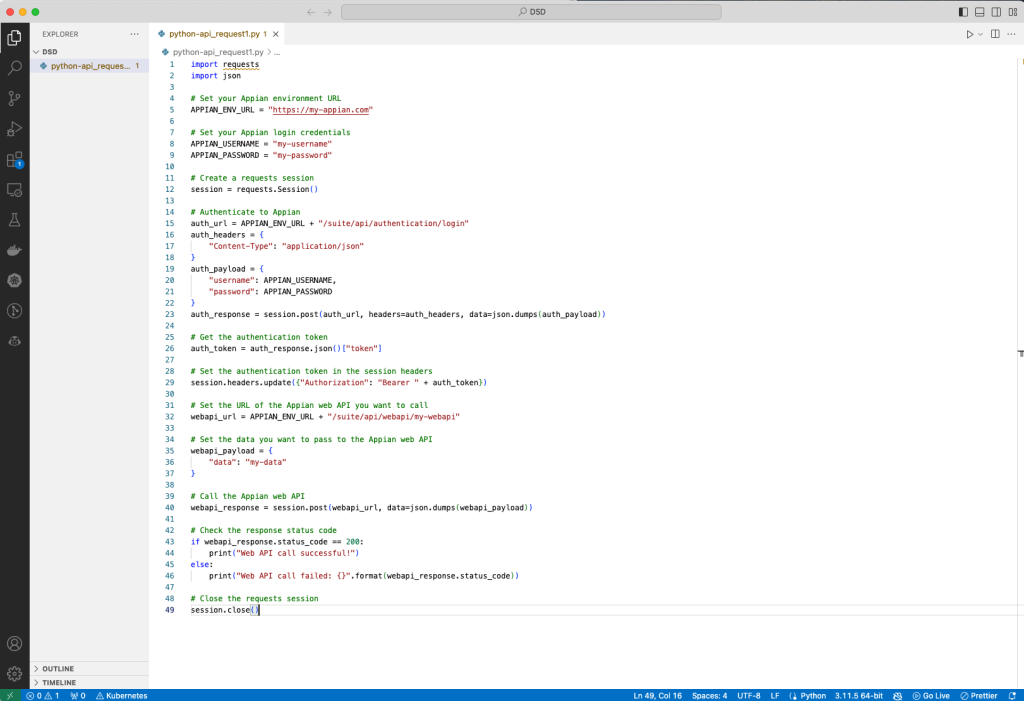