How to read bucket name from AWS SQS Queue using Bash/Shell script:
To read a bucket name from an SQS (Simple Queue Service) message using a Bash script, you’ll need to:
- Receive the SQS message.
- Extract the bucket name from the message.
- Process the bucket name as needed.
Assuming you have AWS CLI configured and the SQS message contains JSON data, you can use the following Bash script as an example:
#!/bin/bash
# Receive the SQS message and store it in a variable
queue_url="YOUR_SQS_QUEUE_URL"
message=$(aws sqs receive-message --queue-url "$queue_url" --max-number-of-messages 1 --wait-time-seconds 20)
# Extract the bucket name from the SQS message
bucket_name=$(echo "$message" | jq -r '.Messages[0].Body' | jq -r '.bucketName')
if [ -n "$bucket_name" ]; then
echo "Bucket Name: $bucket_name"
# Now you can use the $bucket_name variable for further processing
# For example, you can perform operations on the S3 bucket using AWS CLI or other tools.
else
echo "Bucket name not found in the SQS message."
fi
Here’s how the script works:
- It uses the
aws sqs receive-message
command to fetch an SQS message from the specified queue. - It extracts the JSON data from the message.
- It uses
jq
, a lightweight JSON processor, to extract thebucketName
field from the JSON data. - If the bucket name is found, it’s stored in the
bucket_name
variable for further processing.
Make sure to replace YOUR_SQS_QUEUE_URL
with the actual URL of your SQS queue.
Remember that you need to have aws-cli
and jq
installed and properly configured for this script to work. Additionally, you can extend the script to perform actions on the S3 bucket based on the extracted bucket name as needed.
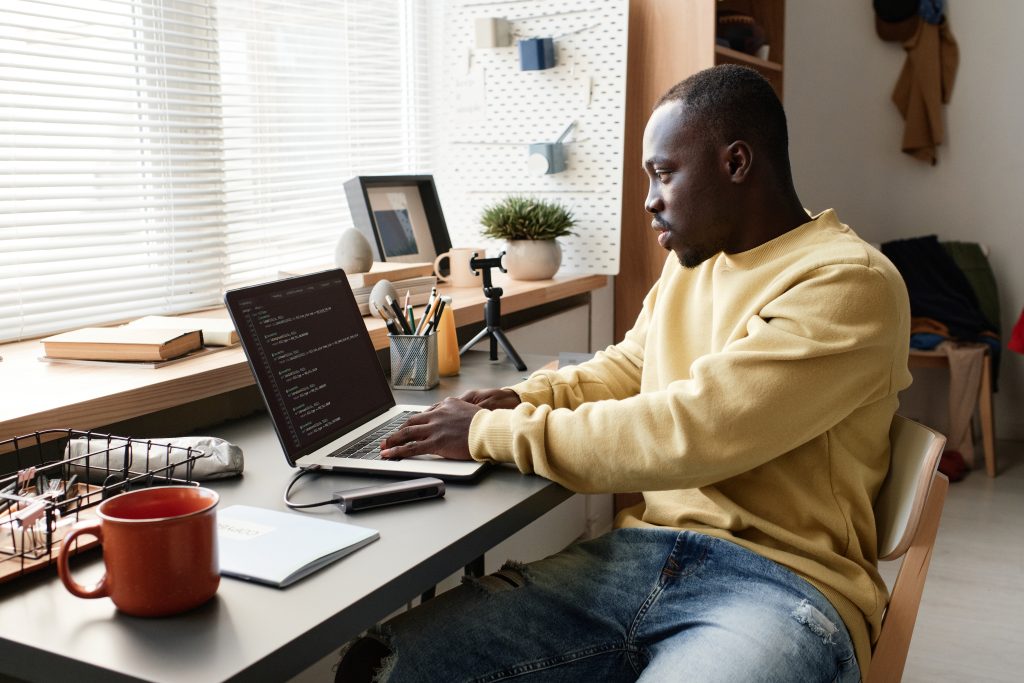
aws-cli
and jq
installed