To check if a file has two extensions in a Bash script, you can use the following approach:
#!/bin/bash
file="example.tar.gz"
# Split the file name into an array using '.' as a delimiter
IFS='.' read -a parts <<< "$file"
# Count the number of parts in the array
num_parts=${#parts[@]}
if [ "$num_parts" -eq 3 ]; then
echo "The file has two extensions."
else
echo "The file does not have two extensions."
fi
In this script:
-
file
contains the filename you want to check. -
IFS='.' read -a parts <<< "$file"
splits the filename into an array using the period (‘.’) as the delimiter. This separates the filename into parts, including the extensions. -
num_parts=${#parts[@]}
calculates the number of parts in the array. - The
if
statement checks if the number of parts is equal to 3. If it is, it means there are two extensions (plus the base filename). If not, it means there are either more or fewer extensions.
You can replace file
with the actual filename you want to check using this script. If the filename has exactly two extensions, it will print “The file has two extensions.” Otherwise, it will print “The file does not have two extensions.”
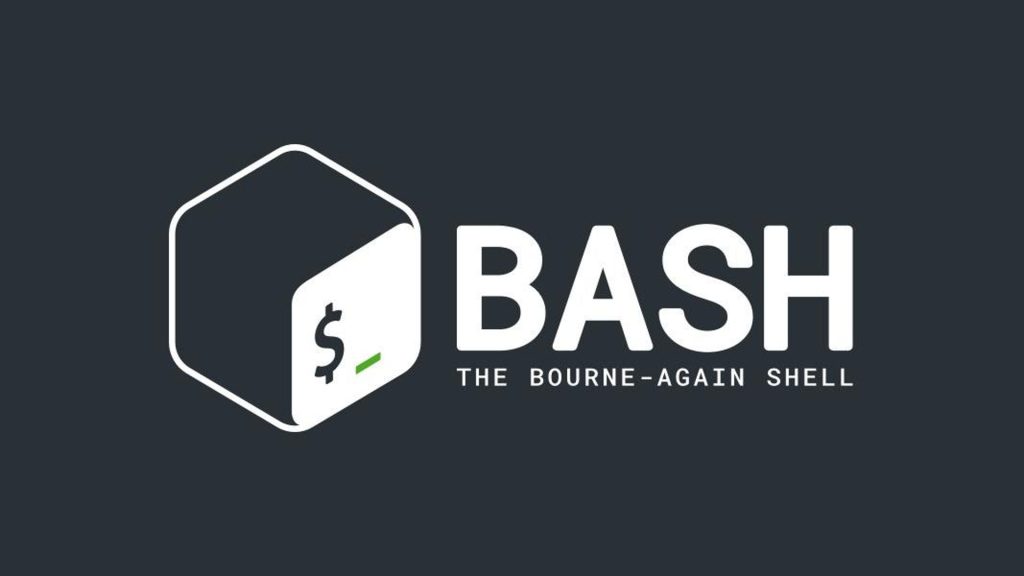