To call an Appian API to pass a value from argv
(command-line arguments) and update the Appian database, you would typically use a scripting language like Python or a tool like cURL. Here’s an example of a Python script that makes an HTTP POST request to an Appian API to update a database with a value from the command line arguments:
import requests
import sys
# Define the Appian API endpoint URL
appian_api_url = 'https://your-appian-api-url.com/update_database_endpoint'
# Get the value from command line arguments (argv)
if len(sys.argv) < 2:
print("Usage: python update_appian.py <value_to_update>")
sys.exit(1)
value_to_update = sys.argv[1]
# Define the data to send in the request body (usually in JSON format)
data = {
"valueToUpdate": value_to_update
}
# Define any headers, such as authentication headers, if needed
headers = {
'Authorization': 'Bearer your_access_token',
'Content-Type': 'application/json'
}
# Make an HTTP POST request to update the database
try:
response = requests.post(appian_api_url, json=data, headers=headers)
# Check the response status code
if response.status_code == 200:
print("Database update successful.")
else:
print(f"Failed to update database. Status code: {response.status_code}")
print(response.text)
except requests.exceptions.RequestException as e:
print(f"Error making API request: {str(e)}")
In this script:
- Replace
'https://your-appian-api-url.com/update_database_endpoint'
with the actual URL of your Appian API endpoint for updating the database. - Ensure you have the necessary authentication mechanism in place, such as an access token, and replace
'your_access_token'
with the actual access token. - The script expects the value to update as a command-line argument. You can run the script like this:
python update_appian.py <value_to_update>
. Make sure to replace<value_to_update>
with the actual value you want to pass to the Appian API. - The
requests
library is used to make the HTTP POST request to the Appian API, and the response is checked for success or failure. - Handle any errors or exceptions that may occur during the API request.
Make sure you have the requests
library installed in your Python environment by running pip install requests
if it’s not already installed.
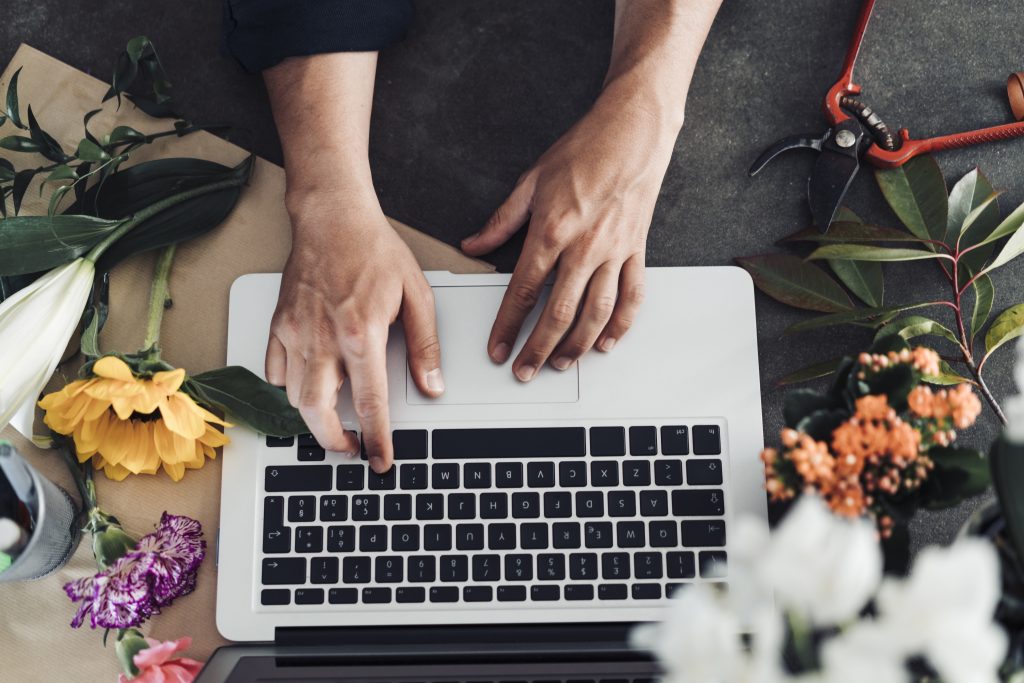
requests
library installed in your Python environment by running pip install requests
if it’s not already installed.